100 Days of SwiftUI
I decided to learn iOS development a couple of months ago. Then, I started checking for online courses from apple's official course and some courses from Udemy. After checking a few lessons, I don't feel right. So, I decided to ask my friend who has been working as an iOS developer and he directed me to check this 100 days of Swift/SwiftUI courses. I checked it and I really liked the flow of the course. So, I have started learning daily and sharing my progress on social media. I will be recording the checklists I have do at times during the course.
Checkpoint 1
- Converting Celcius to Fahrenheit in ios playground
let celcius = 21.0
let fahrenheit = (celcius * 9 / 5) + 32
print("\(celcius)°C = \(fahrenheit)°F")
Output:
21.0°C = 69.8°F
Checkpoint 2
- Create an array of strings, then write some code that prints the number of items in the array and also the number of unique items in the array
let fruits = ["banana", "apple", "orange", "watermalon", "pineapple", "apple", "banana"]
print("Number of fruites: \(fruits.count)")
let uniqueFruits = Set(fruits)
print("Unique fruits: \(uniqueFruits.count)")
Output:
Number of fruites: 7
Unique fruits: 5
Checkpoint 3
- Loop from 1 through 100, and for each number:
- If it’s a multiple of 3, print “Fizz”
- If it’s a multiple of 5, print “Buzz”
- If it’s a multiple of 3 and 5, print “FizzBuzz”
- Otherwise, just print the number.
for i in 1...100 {
let message = "" + (i.isMultiple(of: 3) ? "Fizz" : "") + (i.isMultiple(of: 5) ? "Bizz" : "")
print(message.isEmpty ? i : message)
}
Output:
1
2
Fizz
4
Bizz
Fizz
7
8
Fizz
Bizz
11
Fizz
13
14
FizzBizz
16
17
Fizz
19
Bizz
Fizz
22
23
Fizz
Bizz
26
Fizz
28
29
FizzBizz
31
32
Fizz
34
Bizz
Fizz
37
38
Fizz
Bizz
41
Fizz
43
44
FizzBizz
46
47
Fizz
49
Bizz
Fizz
52
53
Fizz
Bizz
56
Fizz
58
59
FizzBizz
61
62
Fizz
64
Bizz
Fizz
67
68
Fizz
Bizz
71
Fizz
73
74
FizzBizz
76
77
Fizz
79
Bizz
Fizz
82
83
Fizz
Bizz
86
Fizz
88
89
FizzBizz
91
92
Fizz
94
Bizz
Fizz
97
98
Fizz
Bizz
Checkpoint 4
Write a function that accepts an integer from 1 through 10,000, and returns the integer square root of that number. That sounds easy, but there are some catches:
- You can’t use Swift’s built-in
sqrt()
function or similar – you need to find the square root yourself. - If the number is less than 1 or greater than 10,000 you should throw an “out of bounds” error.
- You should only consider integer square roots – don’t worry about the square root of 3 being 1.732, for example.
- If you can’t find the square root, throw a “no root” error.
func findSqrt(of number: Int) throws -> Int {
if number < 1 || number > 10_000 {
throw SqrtError.outOfBounds
}
var result = 2
while result * result < 10_000 {
if result * result == number {
return result
}
result += 1
}
throw SqrtError.noRoot
}
for i in 1...10 {
do {
let result = try findSqrt(of: i)
print("Square root of \(i) = \(result)")
} catch {
print("No Square Root!")
}
}
Output:
No Square Root!
No Square Root!
No Square Root!
Square root of 4 = 2
No Square Root!
No Square Root!
No Square Root!
No Square Root!
Square root of 9 = 3
No Square Root!
Checkpoint 5
Your input is this:
let luckyNumbers = [7, 4, 38, 21, 16, 15, 12, 33, 31, 49]
Your job is to:
- Filter out any numbers that are even
- Sort the array in ascending order
- Map them to strings in the format “7 is a lucky number”
- Print the resulting array, one item per line
let luckyNumbers = [7, 4, 38, 21, 16, 15, 12, 33, 31, 49]
luckyNumbers.filter { $0 % 2 == 0 }.sorted().map { print("\($0) is a lucky number") }
Output:
4 is a lucky number
12 is a lucky number
16 is a lucky number
38 is a lucky number
Checkpoint 6
Create a struct to store information about a car, including its model, number of seats, and current gear, then add a method to change gears up or down. Have a think about variables and access control: what data should be a variable rather than a constant, and what data should be exposed publicly? Should the gear-changing method validate its input somehow?
struct Car {
let model: String
let numberOfSeats: Int
private(set) var currentGear: Int = 1
mutating func changeGear(newGear: Int) {
if newGear > 0 && newGear <= 10 {
currentGear = newGear
} else {
print("Gear not valid")
}
}
}
var myCar = Car(model: "Vox Wagon", numberOfSeats: 4)
print(myCar.currentGear)
myCar.changeGear(newGear: 5)
print(myCar.currentGear)
myCar.changeGear(newGear: 11)
Output:
1
5
Gear not valid
Checkpoint 7
Make a class hierarchy for animals, starting with Animal
at the top, then Dog
and Cat
as subclasses, then Corgi
and Poodle
as subclasses of Dog
, and Persian
and Lion
as subclasses of Cat
.
But there’s more:
- The
Animal
class should have alegs
integer property that tracks how many legs the animal has. - The
Dog
class should have aspeak()
method that prints a generic dog barking string, but each of the subclasses should print something slightly different. - The
Cat
class should have a matchingspeak()
method, again with each subclass printing something different. - The
Cat
class should have anisTame
Boolean property, provided using an initializer.
class Animal {
let legs: Int;
init(legs: Int) {
self.legs = legs
}
}
class Dog: Animal {
func speak() {
print("Bark!")
}
}
class Cat: Animal {
let isTame: Bool
init(legs: Int, isTame: Bool) {
self.isTame = isTame
super.init(legs: legs)
}
func speak() {
print("Meow!")
}
}
class Corgi: Dog {
override func speak() {
print("Corgi says: Woof!")
}
}
class Poodle: Dog {
override func speak() {
print("Poodle says: Woooof!")
}
}
class Persian: Cat {
override func speak() {
print("Persian says: Meooow!")
}
}
class Lion: Cat {
override func speak() {
print("Lion says: Grrr!")
}
}
Checkpoint 8
Make a protocol that describes a building, adding various properties and methods, then create two structs, House
and Office
, that conform to it. Your protocol should require the following:
- A property storing how many rooms it has.
- A property storing the cost as an integer (e.g. 500,000 for a building costing $500,000.)
- A property storing the name of the estate agent responsible for selling the building.
- A method for printing the sales summary of the building, describing what it is along with its other properties.
protocol Building {
var rooms: Int { get }
var cost: Int { get }
var agent: String { get }
func saleSummary()
}
struct House: Building {
var rooms: Int
var cost: Int
var agent: String
func saleSummary() {
print("House with \(rooms) rooms for \(cost)$. Contact agent \(agent)")
}
}
struct Office: Building {
var rooms: Int
var cost: Int
var agent: String
func saleSummary() {
print("Office with \(rooms) rooms for \(cost)$. Contact agent \(agent)")
}
}
var house = House(rooms: 3, cost: 19000, agent: "Kathy")
house.saleSummary()
Output:
House with 3 rooms for 19000$. Contact agent Kathy
Checkpoint 9
Write a function that accepts an optional array of integers, and returns one randomly. If the array is missing or empty, return a random number in the range 1 through 100.
func checkpoint(value: [Int]?) -> Int {
value?.randomElement() ?? Int.random(in: 1...100)
}
print(checkpoint(value: nil))
print(checkpoint(value: [1,2,3]))
Output:
43
2
Project 1
WeSplit app helps to divide the cost among people with custom tip percentages.
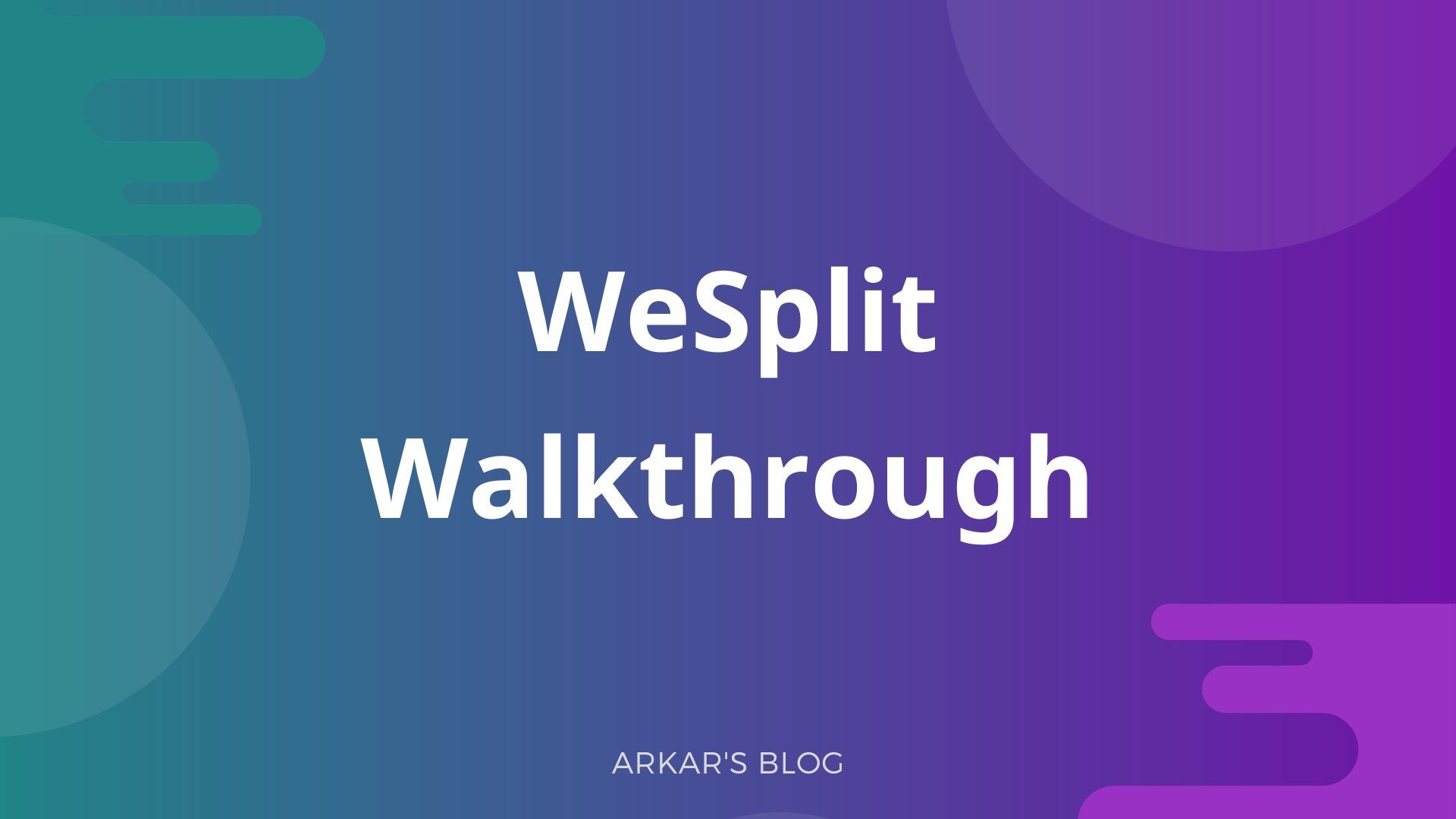
Project 2
Unit Converter app lets you convert the various units for temperature, length, time, and volume.
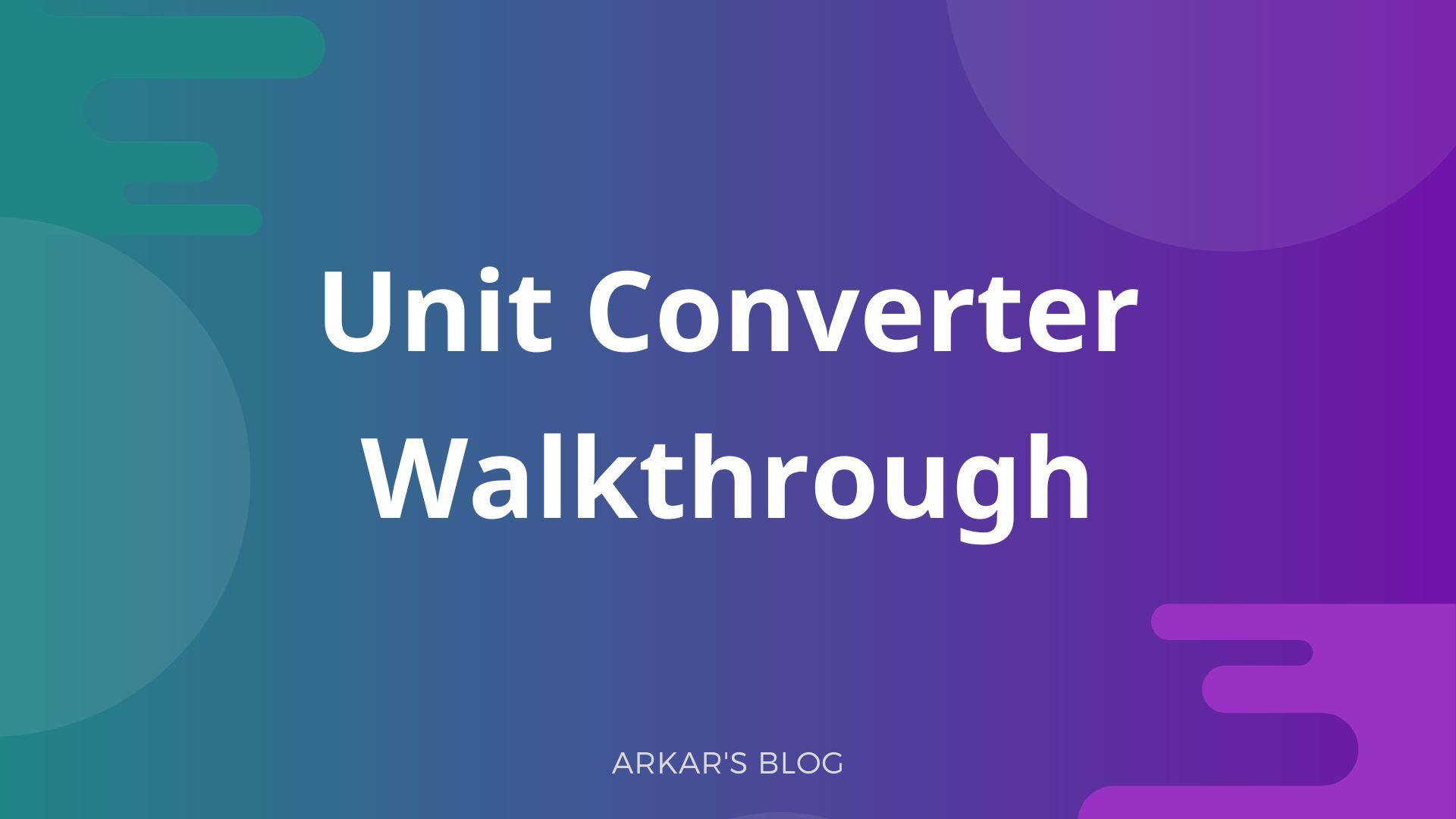
Project 3
Guess the Flag app let you play the game of guessing the flag correctly.
Project 4
Rock Paper Scissor app lets you play the logic game.